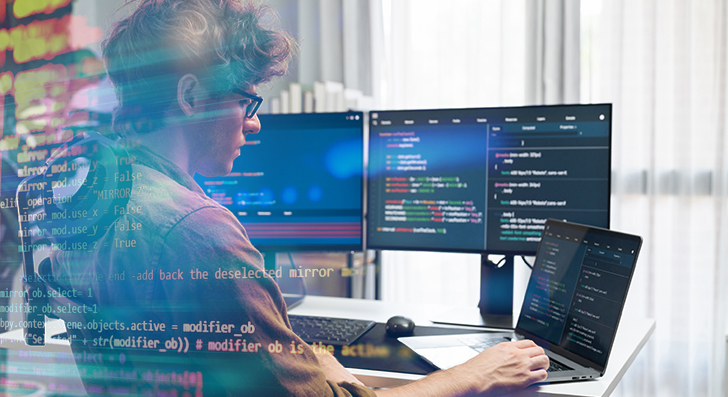
Scalability implies your software can take care of development—more people, far more info, and even more visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and strain later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the Start
Scalability isn't really one thing you bolt on afterwards—it should be section of the plan from the beginning. Many programs are unsuccessful every time they increase quickly for the reason that the initial structure can’t manage the additional load. As being a developer, you'll want to Assume early about how your program will behave stressed.
Begin by coming up with your architecture to be versatile. Stay clear of monolithic codebases exactly where anything is tightly linked. As a substitute, use modular style or microservices. These designs crack your application into smaller, impartial sections. Each module or support can scale on its own with no influencing the whole method.
Also, think of your databases from working day 1. Will it want to take care of a million consumers or maybe 100? Choose the proper form—relational or NoSQL—based on how your info will improve. Program for sharding, indexing, and backups early, Even though you don’t have to have them yet.
An additional crucial level is in order to avoid hardcoding assumptions. Don’t produce code that only will work less than present situations. Take into consideration what would come about When your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design designs that support scaling, like information queues or occasion-driven methods. These assist your app deal with much more requests with no obtaining overloaded.
Once you Construct with scalability in mind, you're not just preparing for success—you might be lessening future headaches. A properly-prepared technique is simpler to take care of, adapt, and improve. It’s superior to get ready early than to rebuild later on.
Use the Right Databases
Choosing the right databases can be a crucial A part of setting up scalable apps. Not all databases are developed the identical, and utilizing the Improper one can sluggish you down and even cause failures as your application grows.
Begin by understanding your facts. Could it be highly structured, like rows in a very desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely robust with interactions, transactions, and consistency. In addition they help scaling techniques like browse replicas, indexing, and partitioning to deal with more website traffic and information.
In the event your info is a lot more flexible—like consumer activity logs, merchandise catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, take into account your study and generate patterns. Will you be doing a lot of reads with much less writes? Use caching and skim replicas. Are you currently dealing with a major create load? Investigate databases which can take care of superior write throughput, and even celebration-centered data storage techniques like Apache Kafka (for short term facts streams).
It’s also good to Believe forward. You might not have to have Sophisticated scaling characteristics now, but deciding on a databases that supports them means you won’t require to switch later.
Use indexing to speed up queries. Stay clear of unnecessary joins. Normalize or denormalize your information based on your accessibility patterns. And usually check database efficiency while you expand.
Briefly, the right database depends on your application’s composition, velocity desires, And just how you assume it to increase. Just take time to choose properly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, each and every little delay provides up. Inadequately composed code or unoptimized queries can decelerate efficiency and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove anything at all pointless. Don’t pick the most intricate Remedy if a straightforward just one operates. Keep your capabilities quick, concentrated, and straightforward to test. Use profiling resources to find bottlenecks—sites the place your code requires much too prolonged to run or works by using excessive memory.
Next, check out your database queries. These generally gradual items down much more than the code by itself. Make sure Every single query only asks for the information you truly want. Avoid Decide on *, which fetches everything, and as a substitute decide on specific fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, especially across significant tables.
Should you detect exactly the same knowledge remaining asked for many times, use caching. Shop the outcome quickly using equipment like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional efficient.
Remember to check with massive datasets. Code and queries that get the job done great with 100 records may crash whenever they have to take care of one million.
In short, scalable apps are quick apps. Keep your code restricted, your queries lean, and use caching when necessary. These methods enable your software keep clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your app grows, it has to handle more users and much more visitors. If every thing goes by means of a single server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching come in. Both of these resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server accomplishing all the do the job, the load balancer routes people to diverse servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused quickly. When people request the same facts once again—like a product site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near to the user.
Caching lowers databases load, improves speed, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally make sure your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are straightforward but impressive resources. Alongside one another, they help your app tackle much more end users, continue to be quick, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that permit your app develop simply. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t have to purchase hardware or guess long term capability. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or mechanically working with vehicle-scaling. When website traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability applications. You could deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, settings—into a person device. This causes it to be effortless to move your application involving environments, from the laptop into the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of multiple containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If just one element of your application crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy conveniently, and Recuperate quickly when challenges occur. In order for you your app to increase without limitations, get started making use of these instruments early. They conserve time, lessen risk, and allow you to continue to be focused on creating, not correcting.
Monitor Almost everything
For those who don’t keep track of your software, you won’t know when items go Erroneous. Checking assists you see how your application is accomplishing, spot problems early, and make greater conclusions as your application grows. It’s a important Portion of making scalable systems.
Commence by monitoring primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and expert services are doing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this facts.
Don’t just watch your servers—observe your application too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for significant complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This aids you repair problems Gustavo Woltmann blog fast, often right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and see a spike in errors or slowdowns, you may roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the proper applications, you are able to Make apps that increase effortlessly with out breaking stressed. Start smaller, think huge, and Establish intelligent.